How to Easily Create a RESTful API for Tests Using json-server
Over the course of the software development process, situations that require an unavailable are very common. That can happen, for example, because of the development environment or because the missing API is under development.
The need to set up a fake API at the very beginning of the project to create mock data or simulate the existence of the original API is very usual, mainly for front-end developers or in the mobile environment. There are also the POC (proof of concept) scenarios in which it’s necessary to simulate the API to expedite the creation process.
When developers do not use the right tools, setting up an API can be a slow and time-consuming, and, therefore, unfeasible process.
JSON-SERVER
json server (the project’s github) is a library in Node.js used to create a fake RESTful API based only on a .json file as the database. And the best is that the library manages the data persistence aspect in this same file.
The library is expected to do that in only 30 seconds (not including npm install) and, actually, that’s possible. The file with the data is a simple structure of objects in json where each property represents an entity of the API, and its value is an array with the available data.
See a simple example:
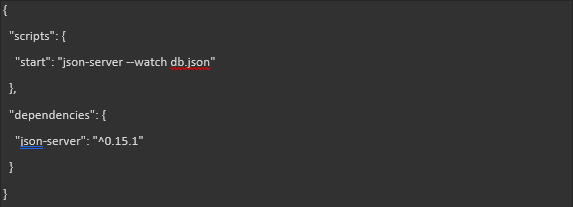
db.json, file with fake API data.
After that the data file is created, you should set up the package.json file as a dependency and create a script to start your project smoothly.
Note: we used the 0.15.1 version, the latest available version at the date this article was written.
package.json:

package.json, with dependencies and startup.
In the folder, use the following command to install the dependencies:
npm install
And the following command to start the API:
npm start
After that, access http://localhost:3000 and you are done!

Home page of our API at http://localhost:3000
Now that the RESTful API is ready, you can use the basics of a REST API to access and change data. It is important to highlight that actions such as PUT, POST and DELETE will have a direct impact on the file.
Here are some examples:
GET http://localhost:3000/posts – Lists all posts
GET http://localhost:3000/posts/1 – Selects the post with ID 1
GET http://localhost:3000/posts?author=douglas – Filters the post based on the field.
GET http://localhost:3000/posts/1/comments – Selects all comments related to the post with ID 1 via the postId file.
POST http://localhost:3000/posts – Creates a new post in the db.json file.
So now you can easily create a RESTful API and speed up your development work, in addition to creating the POC you have been postponing because you did not have an API.
For the example used in this article, see this repository in github.
For more information, see json-server’s official documentation.